Linked List - Whole Explanation
- Krunal Chandan
- Dec 17, 2022
- 3 min read
Updated: Dec 24, 2022
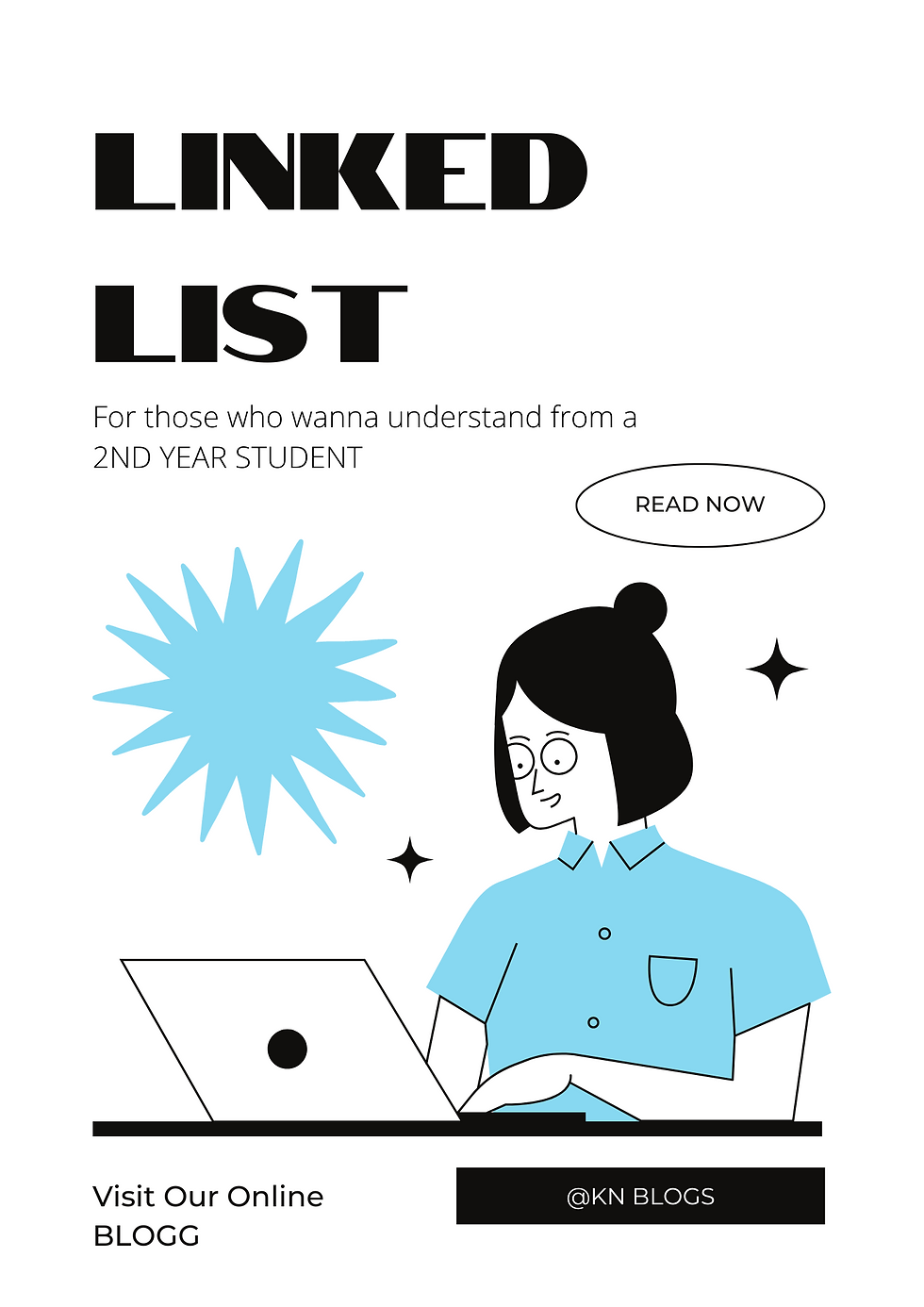
Well it was been told to me that, in last blog, you were told that Linked List will be explained to all. So without delay let's get started. Earlier I was little confused about the topic that how to join those two nodes, or to insert a new node in between 2 nodes or else how to delete any node between any two nodes. Here now I have learned something that can give us a step ahead to these problems.
Well to add a new node we have to construct a new node, by following some of the following code, i.e.,
struct node
{ int data;
struct node *link;
struct node *info;
}
So here we can see, we have created a new node that has two fields i.e., Info and Link. To be specific I have discussed these fields in my previous blog, make sure to read it. Well the info contains the data of the node and the link contains the link or the address of another node, probably like treasure hunt the clue leads to another clue, just in the same case it leads to another node that we have access to or we have stored data to.
To understand better you have to visualize better,
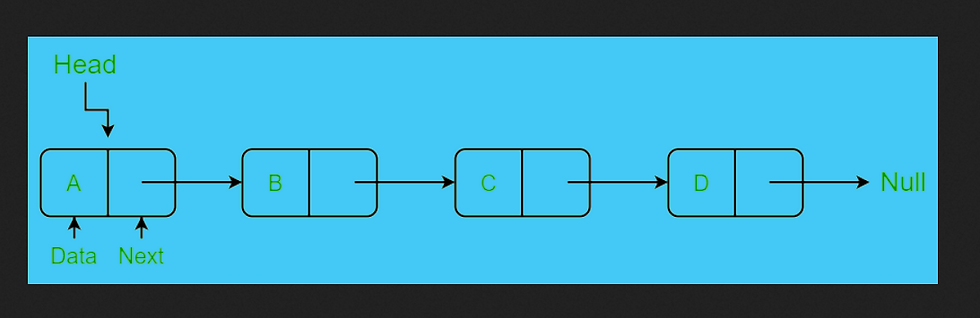
So as here we can see that there are 4 nodes that are connected to each other by links, thus the other field, is containing the data or as we have assigned that is the info. Well the very element or node has it's link given to a different node i.e., Head or Start, both are correct, and the last element or the node has it's link as the NULL, this is so that it should get terminated there and should not get a garbage value, and should not get a infinite loop of nodes.
But the main problem, now is that how do we access the linked list and stores data according to the user or as we can say how to allocate the data to the nodes dynamically, or through runtime. Well to do that we just have to put a for loop, that will simultaneously generates a new node for every new node user wants to enter. And for that we will take from user how many nodes he want to create so that we can give our for loop it's range.
Below is the code you can use to store data in link list,
Before that, you need to allocate memory dynamically to the node you are creating so that it can store something. and to do that, just write these lines of code in the programming console.
newnode = (struct node*) malloc(sizeof(struct node));
Where, Malloc means Memory Allocation, in the heap, it is where we stores the data dynamically.
sizeof() : this refers to the size of the new node, that it should be equal to the others.
Also to store more like this, use a for loop and first of all create a new node that will handle two fields, each. One containing the data and the other the link of the other node or the link of the forward node.
Code like following to store the elements in the linked list,
for( int i = 2; i < (length of node) - 1; i++)
{
// Create a new node
newnode = (struct node*)malloc(sizeof(struct node));
// SET tmep link to newnode as
temp → link = newnode;
// Read elements value form the User
// Now set newnode info to data
// set newnode link as NULL, so that it should get any garbage value
and then store the link of new node into temp, so that you can use it later.
Save it and try to run, if there are errors then solve it, whether you like it or not, it is gonna happen.
}
→ So for today I think this is enough , in next blog I think we can start or give a whirl to the topic of stacks and we will try to learn new things about it.
Recent Posts
See AllMy last blogg about gaming from your android, or my other blogs, that I published earlier were not a big hit, not like this website or...
Comments